Modern frameworks like the Windows Presentation Foundation, its relative Silverlight and the upcoming Windows Runtime make it easy to add animations to a program’s graphical user interface. When implemented well, animations subtly improve the user experience and can demonstrate a high level of polish in your application. The Zune client software and Windows Live Messenger both feature beautiful animations – coincidentally, both were written with internal Microsoft frameworks (Iris and ‘DirectUI’, respectively; the second is apparently unrelated to anything in WinRT). The Twitter client MetroTwit shows what can be done in WPF (its interface was inspired by the Zune software and the Metro design language).
There are times, however, when animations may become detrimental to the user experience. Animations may appear choppy on lower-end or older systems (especially with heavy frameworks like WPF), and in general they should be disabled entirely for Remote Desktop Connection sessions (only very fast networks with low latency may be able to handle them). Jossef Goldberg from the Visual Studio team has written about the efforts made to bring the performance of Visual Studio 2010 over remote connections up to par, and while his advice is mainly about WPF, the same principles apply to other frameworks, also.
So, how can we decide whether animations should be disabled or not? The answer will likely vary from project to project, but there are a few things that we can do:
1. Check if the program is running in a remote session
In Win32, use the GetSystemMetrics function to get the SM_REMOTESESSION metric (a non-zero result means it is a remote session). In WPF, use the SystemParameters.IsRemoteSession property in the System.Windows namespace. In Windows Forms, use the SystemInformation.TerminalServerSession property in the System.Windows.Forms namespace.
2. Check if animations are enabled in Windows
Windows Vista introduced many new animations to common controls like buttons (notice the smooth glow effect and compare it to the binary states found in Windows XP). Regrettably this change made most owner-drawn implementations look shoddy since they mostly lack animation (e.g. buttons in Firefox), but that’s a topic for another post.
Anyway, these animations can be switched off in the following dialog (aside: the list box was mercifully increased in size in Windows 7, though the window still isn’t resizable):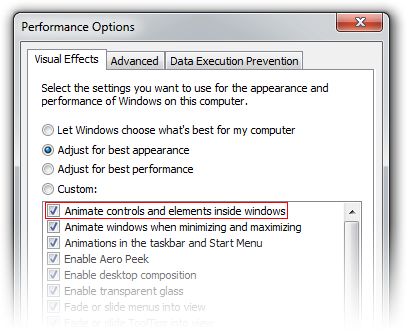
If the user has disabled animations here, it’s probably a good idea to respect that choice in any program you write. We can use the SystemParametersInfo function with the SPI_GETCLIENTAREAANIMATION parameter to check the system setting (or use the SystemParameters.ClientAreaAnimation property in the System.Windows namespace, but note that this always returns true in Windows XP). This only applies to Windows Vista and later.
3. WPF-only: Check the graphics rendering tier
The RenderCapability.Tier property in the System.Windows.Media namespace gives an indication of the graphical capabilities of the system. There are currently 3 tiers, and while the exact meaning may change between .NET releases (as it did in .NET 4.0), this property can be used to help determine what animations, if any, should be enabled.
4. Ask the user!
It can’t hurt to give the user a choice about animations. The remote session checks described above will work for RDP, but there are other technologies available, and we don’t want to punish VNC users by invalidating the entire window 60 times per second for some fancy animation, even if we can’t tell that they’re connecting remotely. If exposing such an option in the UI doesn’t make sense, at least offer it as a command line switch.
Addendum (2011-11-20)
Snooping around PresentationFramework.Aero.dll in .NET Reflector, I happened to come across the ‘Animates’ property in the ButtonChrome class (Microsoft.Windows.Themes namespace):
|
private bool Animates { get { return ((((SystemParameters.PowerLineStatus == PowerLineStatus.Online) && SystemParameters.ClientAreaAnimation) && (RenderCapability.Tier > 0)) && base.IsEnabled); } } |
Similar properties can be found in the other *Chrome classes. Checking the computer’s power status isn’t something covered in this article, but it’s certainly a good idea.